Package json 파일을 서버를 돌릴시 필요하다.
package.json 파일 설치
1. npm init
또는
npm init -y
를 입력시 json 파일 생성
-->
{
"name": "node",
"version": "1.0.0",
"main": "10_console.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC",
"description": ""
}
2. nodemon 설치 (버전도 중요) package.json에 버전이 틀릴시
npm install nodemon --save-dev
또는
npm i nodemon
3. npm i ejs 설치
4. 시작시 npm start 명령어
우선 16_ejs.js 돌릴시 index.js 파일과 index.ejs 파일이 필요하다.
1)
index.js 파일
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>요니의 홈페이지</title>
</head>
<body>
<h2>요니의 홈페이지</h2>
<p><%=name%>님 환영합니다.</p>
<p><a href="./news">뉴스</a></p><p><a href="./favorite.html">즐겨찾기</a></p><p><a href="./resume.html">이력서</a></p>
</body>
</html>
2)
index.ejs 파일
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>요니의 홈페이지</title>
</head>
<body>
<h2>요니의 홈페이지</h2>
<p><%=name%>님 환영합니다.</p>
<p><a href="./news">뉴스</a></p><p><a href="./favorite.html">즐겨찾기</a></p><p><a href="./resume.html">이력서</a></p>
</body>
</html>
3)
16_ejs.js 파일
const http = require('http')
const fs = require('fs')
const ejs = require('ejs')
const name = '김사과'
const jobs = [
{job: '학생'},
{job: '개발자'},
{job: '공무원'},
{job: '취준생'},
{job: '전문직'}
]
const server = http.createServer((req, res) => {
const url = req.url
res.setHeader('Content-Type', 'text/html')
if(url === '/'){
ejs.renderFile('./template/index.ejs', {name:name}).then((data) => res.end(data))
}else if(url === '/news'){
ejs.renderFile('./template/news.ejs', {jobs:jobs}).then((data) => res.end(data))
}
})
server.listen(3000)
pacakge.json에 경로 추가 후 npm start 명령어 실행 후 출력 결과
-->
2.
1)
rest.js 파일
const http = require('http')
const skills = [
{name: 'Python'},
{name: 'MySQL'},
{name: 'HTML'},
{name: 'CSS'},
{name: 'Javascript'},
{name: 'React'},
{name: 'Node.js'},
{name: 'MongoDB'}
]
const server = http.createServer((req, res) => {
res.setHeader('Access-Control-Allow-Origin', '*')
res.setHeader('Access-Control-Allow-Methods', 'GET, POST, OPTIONS')
res.setHeader('Access-Control-Allow-Headers', 'Content-Type')
const url = req.url
const method = req.method
if(method == 'GET'){
res.writeHead(200, {'Content-Type': 'application/json'})
res.end(JSON.stringify(skills))
}
})
server.listen(3000)
실행후 결과
-->
더보기
// 20241109081009
// http://127.0.0.1:3000/
[
{
"name": "Python"
},
{
"name": "MySQL"
},
{
"name": "HTML"
},
{
"name": "CSS"
},
{
"name": "Javascript"
},
{
"name": "React"
},
{
"name": "Node.js"
},
{
"name": "MongoDB"
}
]
3. rest.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>rest</title>
</head>
<body>
<h2>기술 고르기</h2>
<select id="skills">
<option value>기술</option>
<option value>태권도</option>
</select>
<script>
fetch('http://127.0.0.1:3000/')
.then(response => {
if(!response.ok){
throw new error('요청 api가 없음')
}
return response.json()
})
.then(data => {
const skills = document.getElementById('skills')
data.forEach(skill => {
const option = document.getElementById('option')
option.value = skill.name
option.textContent = skill.name
skills.appendChild(option)
})
})
.catch(error => {
console.error('fetch 실패!')
})
</script>
</body>
</html>
결과 화면
-->
더보기
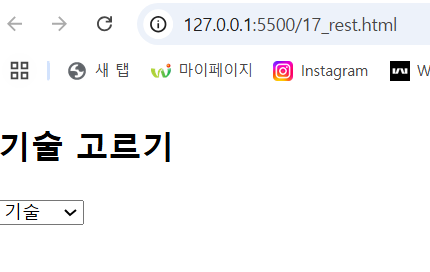
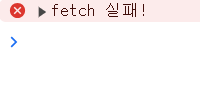
http://127.0.0.1:5500/17_rest.html로 port가 다르므로 오류
Alt + L + O
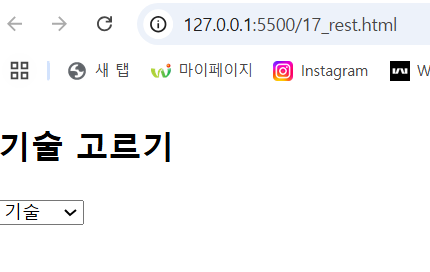
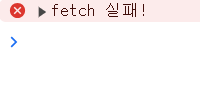
4. EXpress.js
EXpress 웹 프레임워크란?
- 웹서버를 생성하고 HTTP 요청에 대한 라우팅 및 처리, 미들웨어를 통한 요청 및 응답 처리 등을 간단하게 구현할 수 있음
- 다양한 확장 기능과 모듈을 제공하여 개발 생산성을 높일수 있음
(npm i express 설치)
/*
EXpress 웹 프레임워크
- 웹서버를 생성하고 HTTP 요청에 대한 라우팅 및 처리, 미들웨어를 통한 요청 및 응답 처리 등을 간단하게 구현할 수 있음
-다양한 확장 기능과 모듈을 제공하여 개발 생산성을 높일수 있음
npm i express 설치
*/
import express from 'express'
const app = express()
app.use((req, res, next) => {
res.setHeader('node-msg', 'Hi node.js')
next()
})
app.get('/', (req, res, next) => {
res.send('<h2>express 서버로 만든 첫번째 페이지</h2>')
next()
})
app.get('/hello', (req, res, next) => {
res.setHeader('content-type', 'application/json')
res.status(200).json({userid:'apple', name: '김사과', age:20})
next()
})
app.listen(3000)
결과 화면
-->
더보기
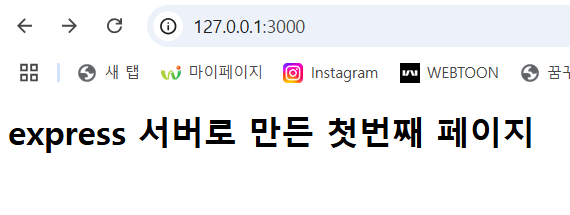
http://127.0.0.1:3000/ 입력시
send('<h2>express 서버로 만든 첫번째 페이지</h2>')
출력
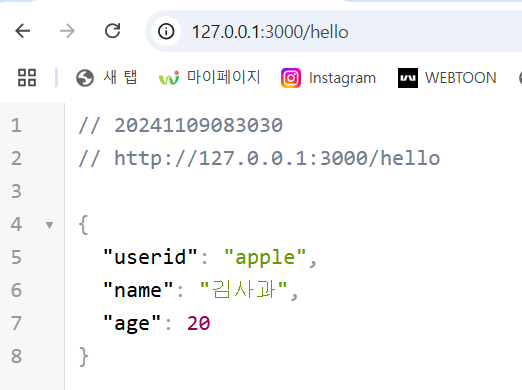
http://127.0.0.1:3000/hello 입력 시
json({userid:'apple', name: '김사과', age:20})
출력
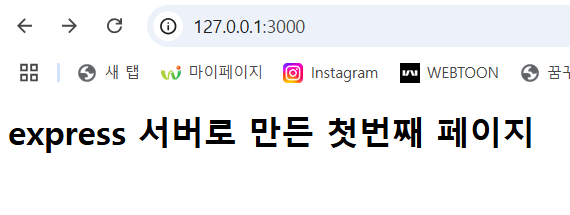
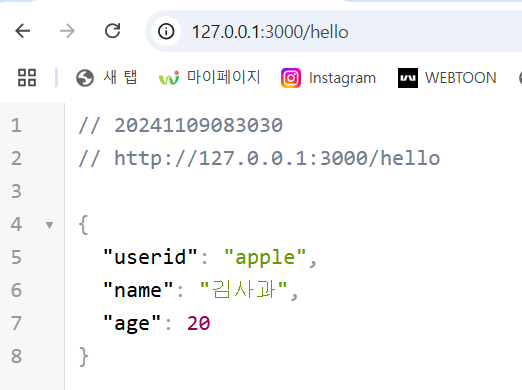
5. Get.js
import express from 'express'
const app = express()
app.get('/', (req, res) => {
res.send('<form action="/login" method="get"><p>아이디: <input type="text" name="userid"></p><p>비밀번호 : <input type="password" name="userpassword"></p><p><button type="submit">로그인</button></p><p><a href="http://127.0.0.1:3000/posts?id=1&userid=apple&name=김사과">클릭</a></p></form>')
})
//http://127.0.0.1:3000/posts
//http://127.0.0.1:3000/posts?id=1
//http://127.0.0.1:3000/posts?id=1&userid=apple&name=김사과
//http://127.0.0.1:3000/posts
app.get('/posts', (req, res) => {
console.log('posts 호출') //post 호출
console.log('path : ', req.path) // path : /posts
console.log('query :', req.query) // query : {}
res.sendStatus(200)
})
//http://127.0.0.1:3000/posts/1
//http://127.0.0.1:3000/posts/1?idx=10
app.get('/posts/:id', (req, res) => {
console.log('posts/ : id 호출') //posts/ : id 호출
console.log('path : ', req.path) // path : /posts/1
console.log('query :', req.query) //query : {}
console.log('params :', req.params) //params : { id: '1' }
res.sendStatus(200)
})
//http://127.0.0.1:3000/member/10
app.get('/member/:userid', (req, res) => {
console.log('req.path :', req.path) //req.path : /member/10
console.log(`${req.params.userid}` , '번 멤버가 출력됨') //10 번 멤버가 출력됨
res.sendStatus(200)
})
/*
로그인 페이지를 이용하여 입력한 id 와 pw를 콘솔에 출력
*/
//http://127.0.0.1:3000/member/userid=10&userpw='김사과'
app.get('/login', (req, res) => {
console.log('login 호출') //login 호출
console.log(req.query) // { userid: 'apple', userpassword: '1234' }
console.log('아이디 : ', req.query.userid) //아이디 : apple
console.log('비밀번호 : ', req.query.userpassword) //비밀번호 : 1234
res.status(200).send('로그인 되었습니다.') //로그인 되었습니다.
//res.sendStatus(200).send('로그인 되었습니다.')
})
app.listen(3000)
결과 화면
-->
더보기
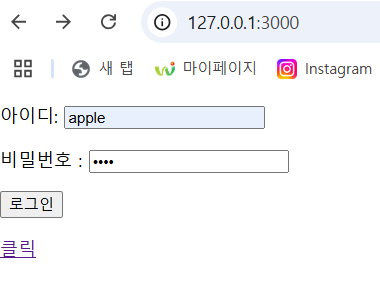
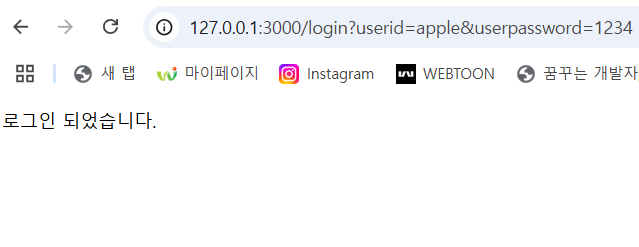
아이디 : apple
비밀번호: 1234
입력시
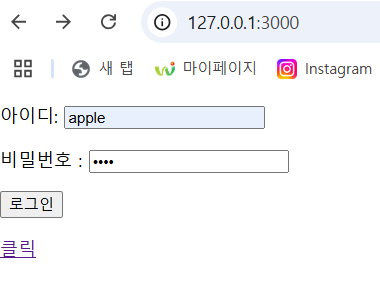
로그인 클릭 시
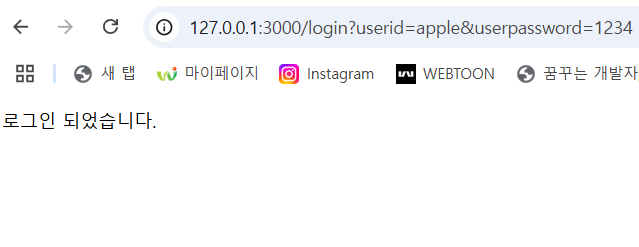
728x90
LIST
'Node.js' 카테고리의 다른 글
Node.js의 로직 순서 (0) | 2024.11.12 |
---|---|
Node.js 활용2 (0) | 2024.11.11 |
Node.js POST 방식 (0) | 2024.11.11 |
2. Node.js 활용 (0) | 2024.11.08 |
Node.js 사용법 (10) | 2024.11.07 |